Welcome to our blog.

Prisma and PostgreSQL vulnerable to NoSQL injection? A surprising security risk explained
Introduction
Imagine you’re building a blogging web app using Prisma. You write a simple query to authenticate users based on their provided email and password:
1const user = await prisma.user.findFirst({
2 where: { email, password },
3});
Looks harmless, right? But what if an attacker sends password = { "not": "" }
? Instead of returning the User object only when email and password match, the query always returns the User when only the provided email matches.
This vulnerability is known as operator injection, but it’s more commonly referred to as NoSQL injection. What many developers don’t realize is that despite strict model schemas some ORMs are vulnerable to operator injection even when they’re used with a relational database such as PostgreSQL, making it a more widespread risk than expected.
In this post, we’ll explore how operator injection works, demonstrate exploits in Prisma ORM, and discuss how to prevent them.
Understanding Operator Injection
To understand operator injection in ORMs, it’s interesting to first look at NoSQL injection. MongoDB introduced developers to an API for querying data using operators such as $eq
, $lt
and $ne
. When user input is passed blindly to MongoDB's query functions, there exists a risk of NoSQL injection.
Popular ORM libraries for JavaScript started offering a similar API for querying data and now almost all major ORMs support some variation of query operators, even when they don’t support MongoDB. Prisma, Sequelize and TypeORM have all implemented support for query operators for relational databases such as PostgreSQL.
Exploiting Operator Injection in Prisma
Prisma query functions that operate on more than one record typically support query operators and are vulnerable to injection. Example functions include findFirst
, findMany
, updateMany
and deleteMany
. While Prisma does validate the model fields referenced in the query at runtime, operators are a valid input for these functions and therefor aren’t rejected by validation.
One reason why operator injection is easy to exploit in Prisma, is the string-based operators that are offered by the Prisma API. Some ORM libraries have removed support for string-based query operators because they are so easily overlooked by developers and easy to exploit. Instead, they force developers to reference custom objects for operators. As these objects cannot be readily de-serialized from user input, the risk of operation injection is greatly reduced in these libraries.
Not all query functions in Prisma are vulnerable to operator injection. Functions that select or mutate a single database record typically do not support operators and throw a runtime error when an Object is provided. Apart from findUnique, the Prisma update, delete and upsert functions also do not accept operators in their where filter.
1 // This query throws a runtime error:
2 // Argument `email`: Invalid value provided. Expected String, provided Object.
3 const user = await prisma.user.findUnique({
4 where: { email: { not: "" } },
5 });
Best Practices to Prevent Operator Injection
1. Cast User Input to Primitive Data Types
Typically casting input to primitive data types such as strings or numbers suffices to prevent attackers from injecting objects. In the original example, casting would look as follows:
1 const user = await prisma.user.findFirst({
2 where: { email: email.toString(), password: password.toString() },
3 });
2. Validate User Input
While casting is effective, you might want to validate the user input, to ensure that the input meets your business logic requirements.
There are many libraries for server-side validation of user input, such as class-validator, zod and joi. If you’re developing for a web application framework such as NestJS or NextJS, they likely recommend specific methods for user input validation in the controller.
In the original example, zod validation might look as follows:
1import { z } from "zod";
2
3const authInputSchema = z.object({
4 email: z.string().email(),
5 password: z.string().min(8)
6});
7
8const { email, password } = authInputSchema.parse({email: req.params.email, password: req.params.password});
9
10const user = await prisma.user.findFirst({
11 where: { email, password },
12});
3. Keep your ORM updated
Stay updated to benefit from security improvements and fixes. For example, Sequelize disabled string aliases for query operators starting from version 4.12, which significantly reduces susceptibility to operator injection.
Conclusion
Operator injection is a real threat for applications using modern ORMs. The vulnerability stems from the ORM API design and isn’t related to the database type in use. Indeed, even Prisma combined with PostgreSQL may be vulnerable to operator injection. While Prisma offers some built-in protection against operator injection, developers must still practice input validation and sanitization to ensure application security.
Appendix: Prisma schema for User model
1// This is your Prisma schema file,
2// learn more about it in the docs: https://pris.ly/d/prisma-schema
3
4generator client {
5 provider = "prisma-client-js"
6}
7
8datasource db {
9 provider = "postgresql"
10 url = env("DATABASE_URL")
11}
12
13// ...
14
15model User {
16 id Int @id @default(autoincrement())
17 email String @unique
18 password String
19 name String?
20 posts Post[]
21 profile Profile?
22}
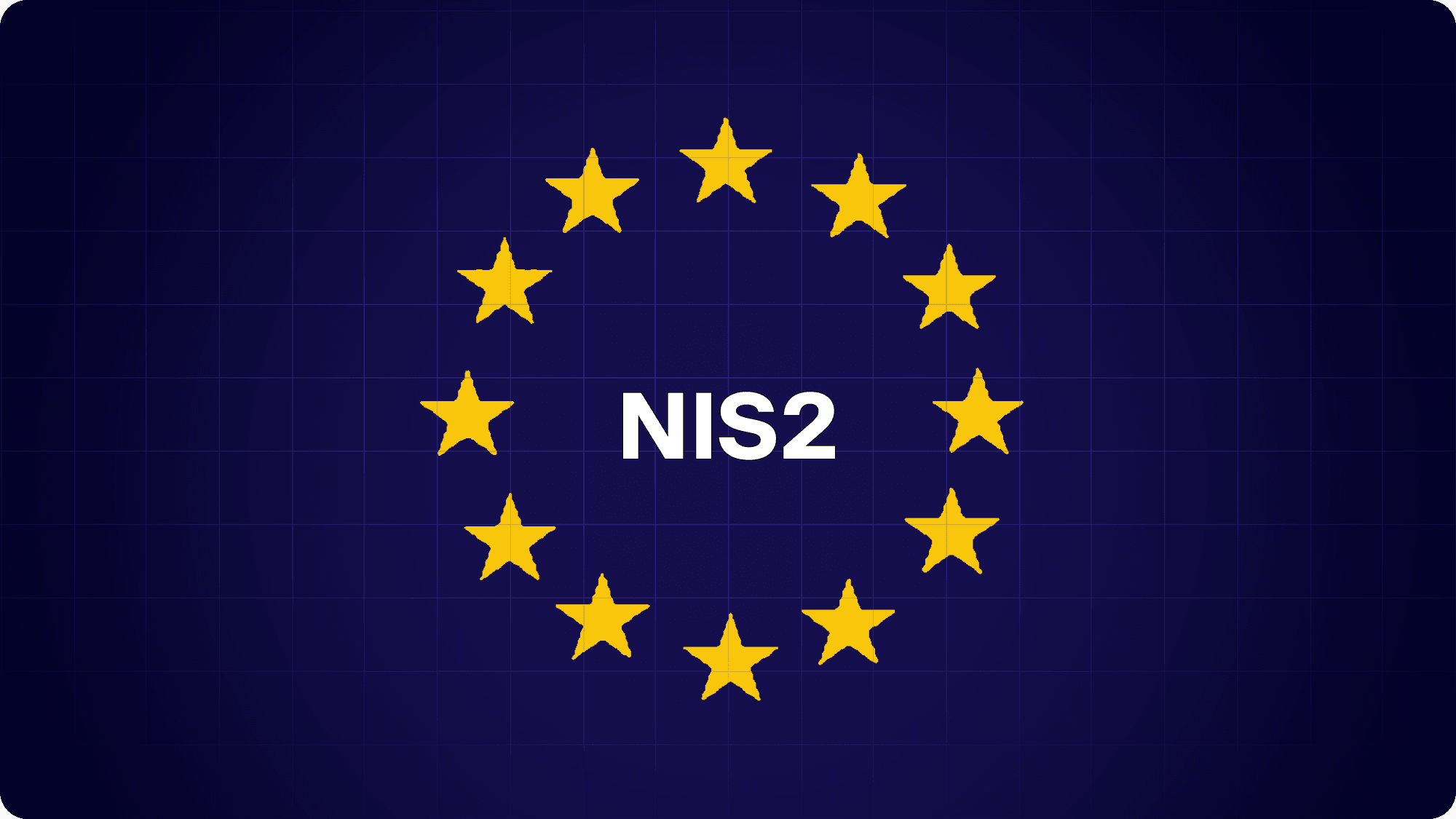
Your Client Requires NIS2 Vulnerability Patching. Now What?
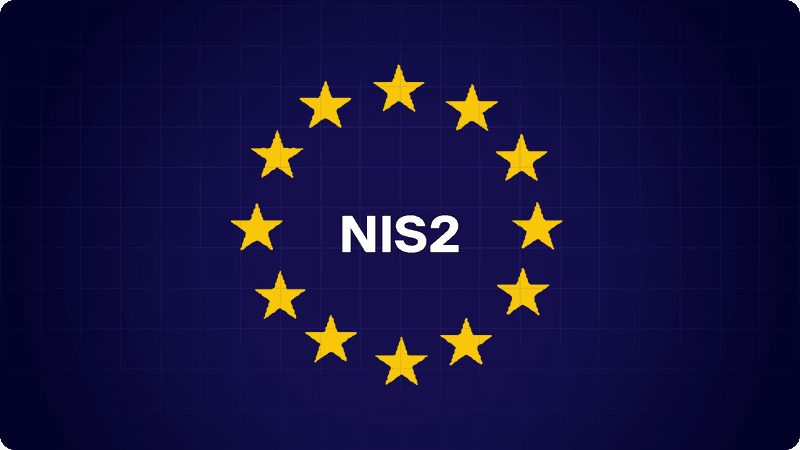
TL;DR: The new EU cybersecurity directive, NIS2, is already reshaping how software suppliers do business through stricter vulnerability management requirements in procurement contracts. This shift is gaining momentum, and more companies will need to adapt. Aikido helps automate compliance reporting and vulnerability tracking to meet these new demands. Start your free compliance journey here, or read on to understand what this means for your business.
The Risks of NIS2 Patching Non-Compliance
Picture this: It's 8:33 AM on a Monday. You're triaging your inbox, coffee in hand, mentally preparing for the weekly meeting at 9. Then you see it – the email subject line that makes your stomach drop.
You open the email, you scroll through the usual boilerplate until you find yourself reading – and re-reading – these words:

All software components used for delivering the services must be patched within the following timeframes, depending on the severity of the vulnerability:
- Critical: within 48 hours of the availability of the patch
- High: within one week of the availability of the patch
- Medium: within one month of the availability of the patch
- Low: within three months of the availability of the patch
48 hours for critical vulnerabilities. Not business days. Not "best effort." 48. Hours. Just like that, your Monday turned into a compliance Olympics, and you're competing in every event simultaneously.
These new NIS2 patching requirements aren't just another checkbox – they present a significant operational challenge. Think about it:
- Your team is already stretched thin
- Every new CVE feels like playing security whack-a-mole
- Your deployment windows are tight and getting tighter
- And now you need to document and prove compliance with these aggressive timelines?
Missing these SLAs isn't just about failing an audit: it could mean losing major contracts, facing penalties, or even being cut out of EU markets entirely.
But here's the thing: while other vendors are scrambling to build massive compliance programs and hiring dedicated NIS2 teams, you don't have to.
How to be NIS2 Compliant
We built Aikido specifically for this moment. Our NIS2 compliance is like playing a game on easy mode for these procurement headaches. In literally minutes, you can:
- Generate compliance reports that procurement teams actually accept
- Track your vulnerability SLAs automatically
- Get alerts before you breach any timelines
- Prove your compliance with actual data, not promises
Let's break down exactly what these requirements mean and how you can tackle them without upending your entire 2025 plan.
Sign up for Aikido and get your free NIS2 report in minutes.
What are the Requirements of NIS2?
NIS2 is the EU's latest cybersecurity directive, and it's reshaping how companies handle vulnerability management. EU Member States are transposing NIS2 into national law, often referencing standards like ISO 27001 as a basis for implementation.
Unlike its predecessor, NIS2 covers more industries and imposes stricter requirements – especially around supply chain security. Major enterprises, particularly those in critical sectors, aren't just implementing these requirements internally; they're required to push them down to every vendor in their supply chain.
Read: NIS2: Who is affected?
What does this mean in practice? If you're selling software or services to EU companies, you'll increasingly face procurement requirements that look exactly like the example above. They'll demand specific patch timelines, expect detailed documentation of your vulnerability management process, and require regular compliance reporting. These aren't just checkbox requirements – procurement teams are actively verifying compliance and building these SLAs into contracts.
The most common requirements we're seeing include:
- Defined SLAs for patching vulnerabilities based on severity (the example in the introduction comes from a real procurement doc!)
- Regular vulnerability scanning and reporting
- Documented processes for vulnerability management
- Evidence of compliance through automated tracking
- Regular status updates on remediation efforts
NIS2 Vulnerability Patching Requirements
Let's cut through the legal jargon and focus on what NIS2 actually implies for vulnerability patching. NIS2 itself doesn't prescribe specific patching timeframes. However, it mandates risk management measures, including vulnerability handling and disclosure, which leads to the described SLAs being imposed by software buyers. Here's what procurement teams are looking for:
Response Timeframes
Most enterprises are standardizing on these patch windows:
- Critical vulnerabilities: 48 hours
- High severity: 7 days
- Medium severity: 30 days
- Low severity: 90 days
And yes, these timelines start from when the patch becomes available, not from when you discover it. This means you need to stay on top of vulnerability announcements for every component in your stack.
Documentation Requirements
You'll need to prove three things:
- When you discovered each vulnerability
- When the patch became available
- When you deployed the fix
Without automated tracking, this quickly becomes a full-time job for your security team.
Continuous Monitoring
Gone are the days of quarterly security scans. NIS2 expects:
- Regular vulnerability scanning (most companies interpret this as daily)
- CVEs monitoring for new security advisories
- Active tracking of patch status and SLA compliance
Risk Management
For every vulnerability, you need to:
- Document your severity assessment
- Track remediation progress
- Justify any delays in patching
- Report on compliance with agreed SLAs
This is why we built the NIS2 report in Aikido – it handles all of this automatically. Instead of building spreadsheets and juggling tickets, you get a single dashboard that tracks everything procurement teams want to see.
Implementing with Aikido (Practical Steps)
Here's the thing about compliance frameworks: they're usually a mess of paperwork disconnected from your actual security operations. But it doesn't have to be that way.
Let's say a procurement team asks about your vulnerability management process. Instead of scrambling to create documentation, you simply:
- Connect Aikido to your development pipeline
- Link your cloud infrastructure
- Enable the NIS2 compliance report
- Export the automated evidence of your patching timelines
- Send it off
That's it. No endless documentation sessions. No spreadsheets. No last-minute panics.
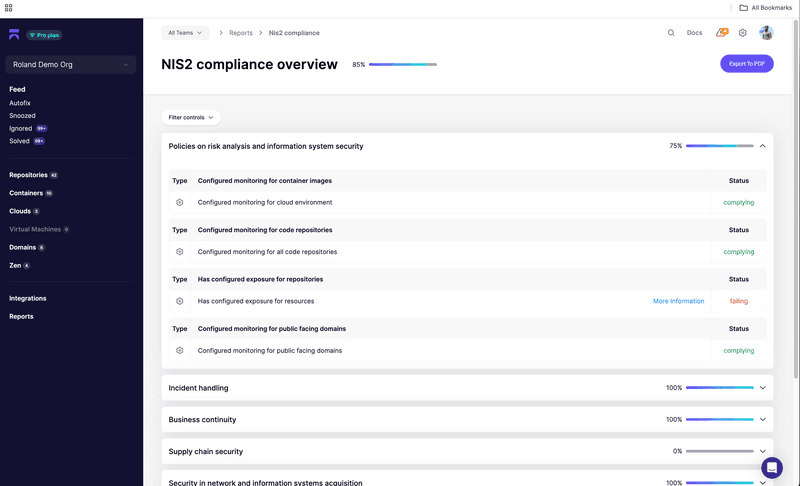
What You Get Immediately
- Continuous scanning across nine risk vectors (from dependencies to runtime security)
- Automated evidence collection for your technical controls
- Real-time compliance status for procurement requirements
- Dynamic reporting that maps directly to NIS2 requirements
The best part? It's working exactly where your developers already are: in CI pipelines, code repositories, and cloud environments. While other teams are manually collecting evidence for their ISO 27001 certifications and NIS2 compliance efforts, you're automatically generating compliance reports from your actual security data.
Next Steps
Let’s wrap up in one sentence: you don't need to build a massive compliance program or hire an army of consultants to answer NIS2 compliance inquiries. We recommend the following strategic approach
- Get a clear picture of where you stand (by signing up for a free Aikido account)
- Run your first NIS2 compliance report
- See exactly what needs attention
- Automate the reporting & easily prove to your customer you're ready for NIS2
While your competitors continue to manage their patch documentation through manual processes, you could have automated compliance reporting up and running in minutes. When future procurement inquiries regarding NIS2 requirements arrive, you can proceed with confidence, knowing your compliance infrastructure is firmly in place.
So the next time you see a procurement email with 'NIS2 requirements' in the subject line? Go ahead and take another sip of that coffee. You've got this one in the bag – or should we say, in the mug.
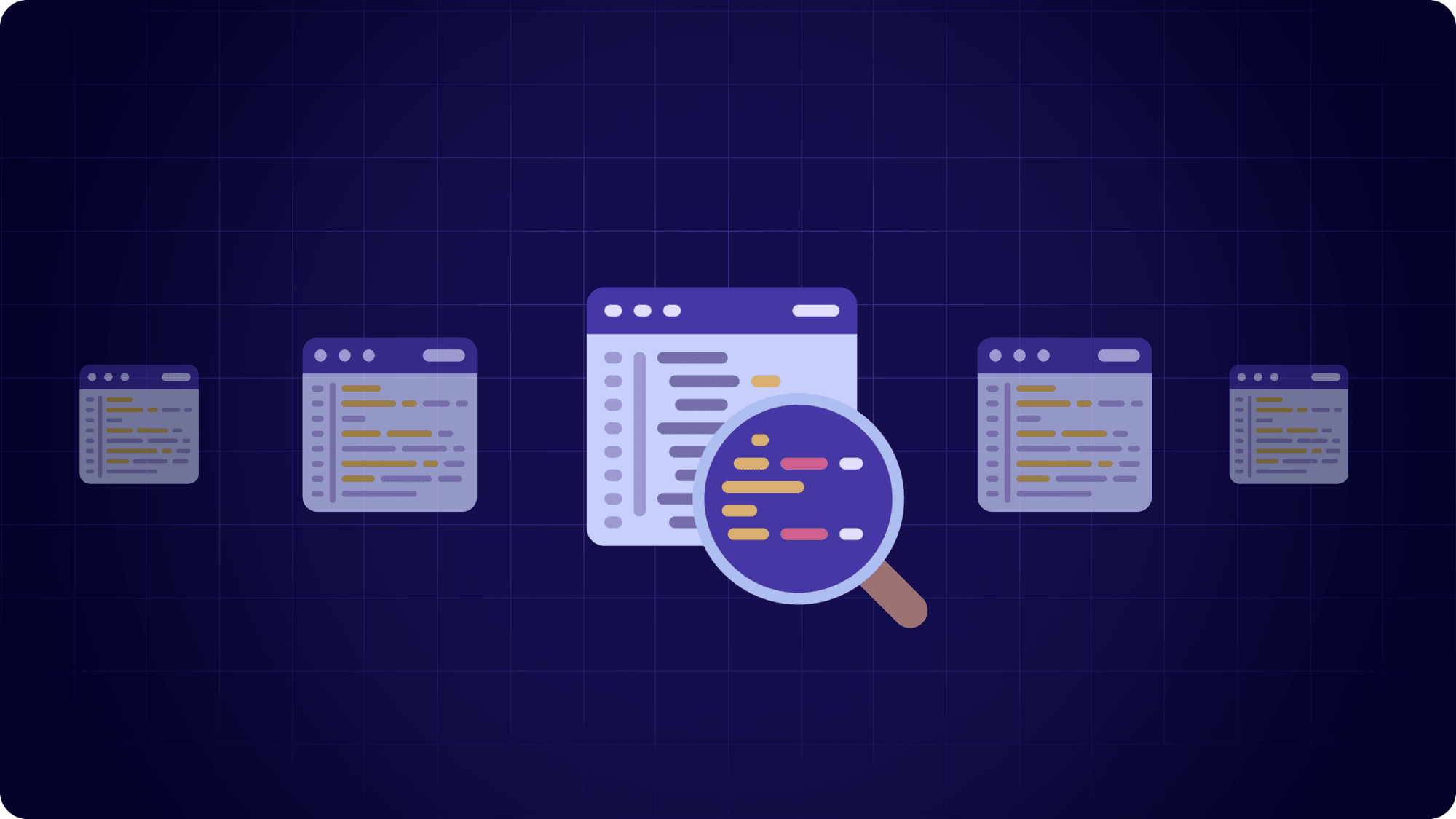
Top 10 AI-powered SAST tools in 2025

In this article, we break down the 10 leaders in AI SAST tools. We explore the core features of each tool and the unique ways they implement AI to enhance security discovery, prioritization and remediation.
What is SAST?
Static Application Security Testing (SAST) is a methodology for analyzing an application's source code, bytecode, or binary to identify vulnerabilities and security flaws early in the software development lifecycle (SDLC). SAST finds vulnerabilities within the source code, which means that it is often the first defense against insecure code.
For more information SAST vs DAST what you need to know
What vulnerabilities does SAST find in your code?
There are many different vulnerabilities SAST can find and it depends on the coding practices used, technology stack and frameworks. Below are some of the most common vulnerabilities a SAST tool will typically uncover.
SQL Injection
Detects improper sanitization of user inputs that could lead to database compromise.
Example Injection Code
# Function to authenticate user
def authenticate_user(username, password):
query = f"SELECT * FROM users WHERE username = '{user}' AND password = '{password}'"
print(f"Executing query: {query}") # For debugging purposes
cursor.execute(query)
return cursor.fetchone()
Cross-Site Scripting (XSS)
Identifies instances where user inputs are incorrectly validated or encoded, allowing injection of malicious scripts.
Example of code vulnerable to XSS
<script>
const params = new URLSearchParams(window.location.search);
const name = params.get('name');
if (name) {
// Directly inserting user input into HTML without sanitization
document.getElementById('greeting').innerHTML = `Hello, ${name}!`;
}
</script>
Buffer Overflows
Highlights areas where improper handling of memory allocation could lead to data corruption or system crashes.
Example code vulnerable to buffer overflow
1#include
2void vulnerableFunction() {
3 char buffer[10]; // A small buffer with space for 10 characters
4
5 printf("Enter some text: ");
6 gets(buffer); // Dangerous function: does not check input size
7
8 printf("You entered: %s\n", buffer);
9}
10
11int main() {
12 vulnerableFunction();
13 return 0;
14}
Insecure Cryptographic Practices
Finds weak encryption algorithms, improper key management, or hardcoded keys.
import hashlib
def store_password(password):
# Weak hashing algorithm (MD5 is broken and unsuitable for passwords)
hashed_password = hashlib.md5(password.encode()).hexdigest()
print(f"Storing hashed password: {hashed_password}")
return hashed_password
SAST tools provide valuable insights, enabling developers to fix issues before they become critical.
How AI is Enhancing SAST Tools
Right now you can’t get away from AI buzz (and BullSh*t). It can be difficult to know exactly how AI is being implemented in security tools. We wanted to compare some of the leaders in AI-powered SAST and explain the different ways these tools are implementing AI to enhance security.
Right now there are three trends with AI as it relates to SAST tools.
- AI to improve vulnerability detection: AI models trained on large datasets of known vulnerabilities improve the accuracy of identifying security issues while reducing false positives.
- AI to create automated prioritization: AI helps rank vulnerabilities based on severity, exploitability, and potential business impact, allowing developers to focus on critical issues first.
- AI to provide automated remediation: AI provides context-aware code fixes or suggestions, speeding up the remediation process and helping developers learn secure coding practices.
Top 10 AI-Powered SAST Tools
Here are 10 industry leaders that are using AI in different ways to enhance the capabilities of traditional SAST (In alphabetical order)
1. Aikido Security SAST | AI AutoFix

Core AI Capability | Auto Remediation (Dashboard + IDE)
Aikido Security uses AI to create code fixes for vulnerabilities discovered by its SAST scanner and can even generate automated pull requests to speed up the remediation process.
Unlike other tools, Aikido does not send your code to a third-party AI model and has a unique method of ensuring your code does not leak through AI models. Aikido creates a sandbox environment of your code, then a purpose-tuned LLM will scan it, and create suggestions which are also scanned again for vulnerabilities. Once the suggested remediation as passed validation, a pull request can be automatically created before finally the Sandbox environment is destroyed. Aikidos AutoFix is also able to give a confidence score on the suggestions it makes to developers to make informed decisions when using AI-generated code.
2. Checkmarx
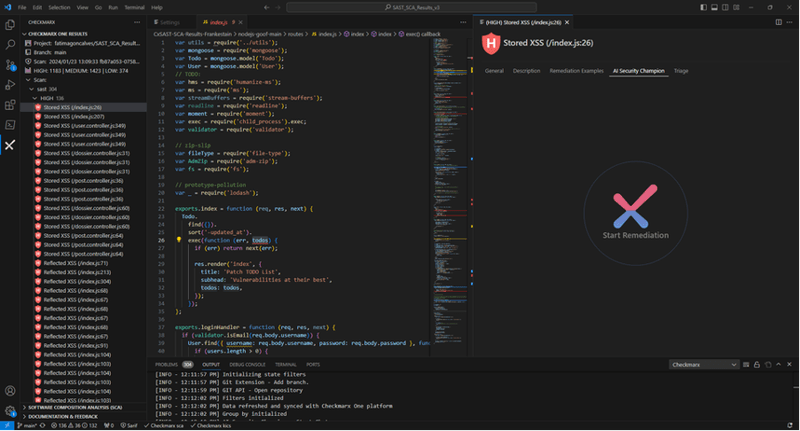
Core AI Capability | Auto Remediation (IDE only)
Checkmarx SAST tools can provide AI-generated coding suggestions to developers within their IDE. The tool connects to ChatGPT transmits the developer's code to the OpenAI model and retrieves the suggestions. This method makes the process of querying ChatGPT easier but does not add any proprietary processes giving it limited capabilities right now.
WARNING - This use-case sends your proprietary code to OpenAI and may not meet compliance standards.
3. CodeAnt AI

Core AI Capability | Improved Detection (Dashboard)
CodeAnt is a code security and quality tool that entirely uses AI for its discovery of code vulnerabilities and suggested fixes. CodeAnt does not provide documentation on how their AI models work but generally uses AI as their core detection engine, this can slow down detection, particularly in large enterprises.
4. CodeThreat
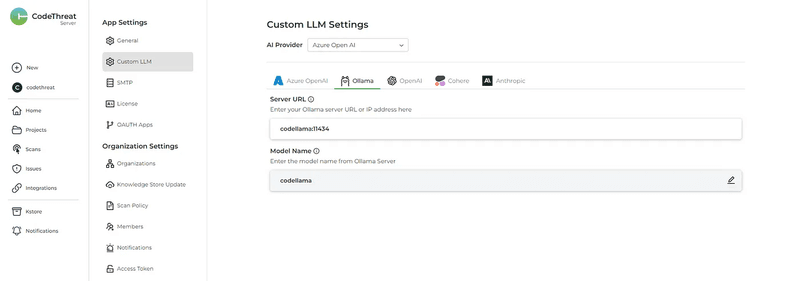
Core AI Capability | Automated Prioritization (Dashboard)
CodeThreat offers on-premise static code analysis and provides AI-assisted remediation strategies. A core difference is that CodeThreat allows you to integrate your own on-premise AI model into their tool. This has the advantage of not sending data to a third party but means it can only offer genetically trained AI models right now and you need to be running an on-premise AI LLM like ChatGPT.
5. Fortify Static Code Analyzer

Core AI Capability | Improved Prioritization (Dashboard)
Fortify Static Code Analyzer scans source code for vulnerabilities and gives users the option to adjust thresholds when an alert is made for example likeliness of exploitability. Fortifies AI Autoassistant reviews the previous thresholds assigned to vulnerabilities and makes intelligent predictions on what the thresholds for other vulnerabilities should be.
Note: Fortify Static Code Analyzer does not use AI to discover vulnerabilities or suggest fixes for them, instead it uses it to predict administrative settings used in the admin panels.
6. GitHub Advanced Security | CodeQL
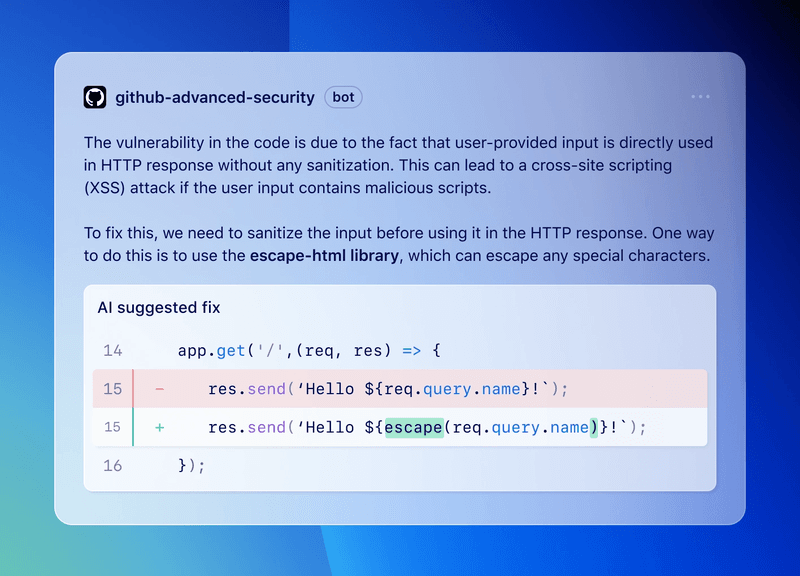
Core AI Capability | Auto Remediation (IDE + Dashboard)
GitHub CodeQL is a static code scanner that uses AI to create intelligent auto-remediation in the form of code suggestions. Developers can accept or dismiss the changes via pull requests in GitHub CodeSpaces or from their machine. It's part of GitHub Advanced Security.
7. Qwiet AI | SAST Code

Core AI Capability | Auto Remediation (Dashboard)
Qwiet AI SAST is a rule-based static application security testing tool that leverages AI to auto-suggest remediation advice and code fixes for code vulnerabilities. Its core offering is its three-stage AI agents which Analyze the issue, Suggest a fix, and then validate the fix.
8. Snyk Code | DeepCode
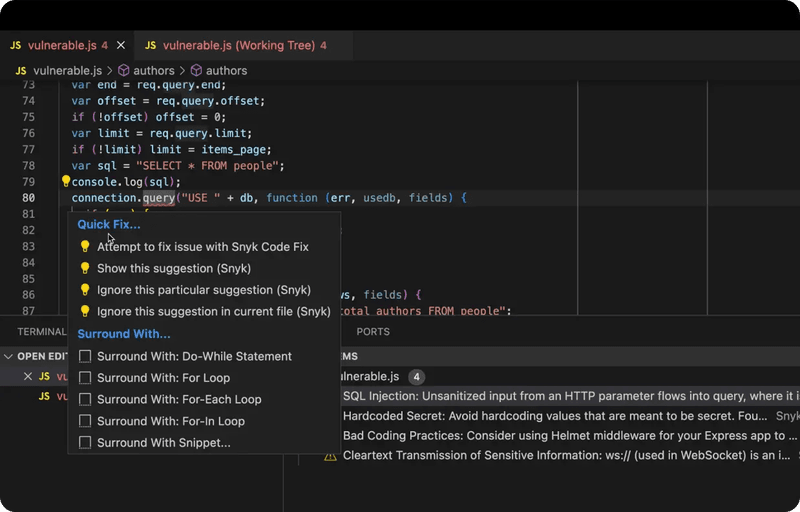
Core AI Capability | Auto Remediation (IDE)
Snyk Code, a developer-focused, real-time SAST tool that can provide code suggestions to developers from within the IDE thanks toDeepCode AI which Snyk acquired. DeepCode AI utilizes multiple AI models and the core selling point is that their models are trained on data curated by top security specialists giving improved confidence in the AI results.
9. Semgrep Code
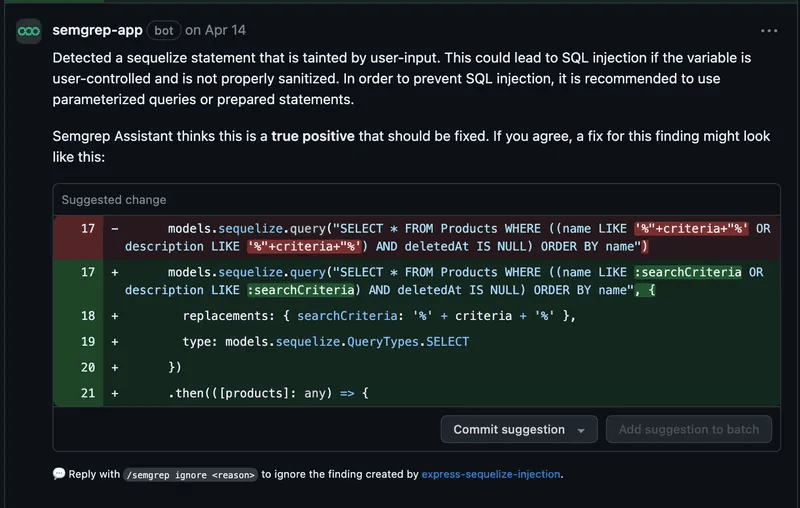
Core AI Capability | Improved Detection
SemGreps AI assistant, aptly named assistant, uses the context of the code surrounding a potential vulnerability to provide more accurate results and provide recommended code fixes. It can also be used to create rules for SemGrep to enhance its detection based on the prompts you provide.
10. Veracode Fix

Core AI Capability | Auto Remediation
Veracode fix uses AI to suggest changes based on vulnerabilities within code when developers are using the Veracode IDE extension or CLI tool. The main differentiator for Veracode Fix is that their custom-trained model is not trained on code in the wild but on known vulnerabilities within their database. The positive of this will be more confidence in suggested fixes, the negative is that it is more limited in the scenarios that it can suggest code fixes.
How to choose a SAST tool
AI) is a relatively new entrant to the security market, and industry leaders are continuously exploring innovative applications. AI should be viewed as a tool to enhance security systems, rather than as a sole source of truth. It's important to note that AI cannot transform subpar tools into effective ones. To maximize its potential, AI should be integrated with tools that already have a robust foundation and a proven track record.

Snyk vs Aikido Security | G2 Reviews Snyk Alternative
So you’re in the market for application security, perhaps even a Snyk alternative. Whether it’s your first time exploring a code security platform or you’re a seasoned user searching for better options, you’re in the right place.
When developers and businesses evaluate their choices, two names often rise to the top: Aikido Security and Snyk. Both platforms offer comprehensive tools for engineering teams to secure their applications, but how do they really compare? Rather than relying on opinions, let’s turn to the voices that matter most: real users.
Based on verified 3rd-party reviews
This guide is a direct synopsis of verified third-party reviews from G2, the world’s largest trusted software marketplace. Over 100 million professionals rely on G2 annually to make informed software decisions using authentic user feedback. Based on the latest verified user data from G2, we’ll provide a detailed breakdown of Aikido Security vs. Snyk, analyzing features, user experience, pricing, and more.
In addition, you can also read these user reviews directly on G2. Here is the G2 link for Aikido Security and for Snyk, and the direct comparison reviews that compare Aikido as a Snyk alternative.
What is Aikido Security:
Led by serial CTO Willem Delbare, Aikido is the “no bullshit” security platform for developers. After many years using other application security products, Delbare founded Aikido to fix security for CTOs and developers with an all-in-one platform code-to-cloud security platform designed to help engineering teams get security done.
Engineering teams execute faster with Aikido thanks to developer-dedicated features: centralized scans, aggressive false positive reduction, dev-native UX, automatic risk triage, risk bundling, and easy step-by-step risk fixes, including LLM-powered autofixes for 3 different issue types.
TL;DR Aikido makes security simple for SMEs and doable for developers, so companies can win customers, grow up-market, and ace compliance.
What is Snyk:
Snyk is a well-known security company that positions itself as a “developer-oriented” security tool, for teams to identify and fix vulnerabilities in their code, open-source dependencies, and container images. Snyk is an early player in the “shift left” security movement and was founded 10 years ago in Tel Aviv and London and is currently headquartered in Boston, USA.
Aikido vs Snyk Alternative at a Glance
- Aikido Security:
- Rating ⭐️: 4.7
- Market Segments: Small to Mid-Market Businesses
- Entry-Level Pricing: Free
- Snyk:
- Rating ⭐️: 4.5
- Market Segments: Mid-Market to Enterprise
- Entry-Level Pricing: Free
Aikido Security is heavily favored by small to medium-sized businesses, while Snyk has broader adoption among larger mid-market organizations, especially enterprises. Both platforms offer free plans, making them accessible for individual developers and smaller teams.
Category Ranking Overview

User Experience
Ease of Use
- Aikido Security: Rated 9.5, users praise its intuitive interface and streamlined workflows. It’s designed with a developer-first approach, ensuring minimal friction when integrating into existing CI/CD pipelines.
- Snyk: Rated 8.7, while still user-friendly, some reviewers note a steeper learning curve, especially for teams unfamiliar with DevSecOps tools.
Ease of Setup
- Aikido Security: With a score of 9.5, users love Aikido’s quick onboarding process and minimal configuration requirements.
- Snyk: Rated 9.0, setup is straightforward, but users occasionally encounter challenges integrating with less common tools.
Ease of Administration
- Aikido Security: Scoring 9.3, system administrators find it simple to manage teams, permissions, and integrations.
- Snyk: Rated 8.8, administration is effective but can become complex in larger organizations.
Support and Product Direction
Quality of Support
- Aikido Security: With an impressive score of 9.6, users frequently commend the responsive and knowledgeable support team. Most testimonials highlight fast support from Aikido team and founders as a top highlight.
- Snyk: Rated 8.6, support is OK, generally reliable but sometimes slower for free-tier users.
Product Direction
- Aikido Security: Users rank Aikido with a round 10.0 score, reflecting user confidence in its innovative roadmap and consistent feature updates.
- Snyk: Rated 8.7, appreciated for its focus on open source and developer-centric tools but slightly lagging in comprehensive feature rollouts.
Aikido vs Snyk Alternative Feature Comparison
If you are looking for a Snyk alternative, it is important to note the specific production functionalities that each platform offers. While Snyk offers SAST, IaC, Software Composition Analysis, and vulnerability scanning, Aikido offers more functions and features within its all-in-one platform.
While Snyk offers 4 products, Aikido offers 11 products in one security suite, including SAST, DAST, Software Composition Analysis, IaC, container image scanning, secret scanning, malware scanning, API scanning, license risk scanning, local custom scanning, as well as cloud (CSPM) security.

Static Application Security Testing (SAST)
What is it? SAST is a method to identify vulnerabilities in source code before deployment.
- Aikido Security: Rated 8.7, it excels in identifying vulnerabilities in source code and presenting actionable insights.
- Snyk: Rated 7.7, effective but often criticized for generating more false positives compared to competitors.
Dynamic Application Security Testing (DAST)
What is it? DAST is a technique that scans live applications to detect runtime vulnerabilities.
- Aikido Security: Scoring 8.9, users appreciate its ability to identify runtime vulnerabilities with minimal configuration.
- Snyk: Not enough data available to assess DAST capabilities.
Container Security
What is it? Container Security is the process of identifying vulnerabilities in containerized applications and images.
- Aikido Security: Rated 8.4, it provides deep insights into container images and vulnerabilities across registries.
- Snyk: Rated 7.9, strong for basic container scanning but less comprehensive in advanced scenarios.
Software Composition Analysis (SCA)
What is it? SCA is the practice of detecting vulnerabilities in open-source dependencies and third-party libraries.
- Aikido Security: Scoring 8.9, it combines open-source dependency scanning with enhanced malware detection, ensuring robust protection.
- Snyk: Rated 8.0, effective for detecting known vulnerabilities in open-source libraries but less advanced in identifying malicious packages.
Application Security Posture Management (ASPM)
What is it? ASPM is a framework for managing and improving the security posture of applications across their lifecycle.
- Aikido Security: Scored 8.4, praised for its proactive approach to identifying and resolving security risks in application environments.
- Snyk: Not enough data available to assess ASPM capabilities.
Cloud Security Posture Management (CSPM)
What is it? CSPM is a toolset for monitoring and securing cloud environments by identifying misconfigurations and compliance issues.
- Monitors and secures cloud environments by identifying misconfigurations and compliance issues.
- Aikido Security: Rated 7.4, integrates seamlessly into multi-cloud environments, providing clear misconfiguration insights. Aikido CSPM functionality was recently launched and a big facet of our roadmap.
- Snyk: Not enough data is available to evaluate CSPM features. At this time, Snyk does not have CSPM functionality.
Vulnerability Scanner
What is it? A Vulnerability Scanner identifies and evaluates security vulnerabilities in systems and software.
- Aikido Security: Rated 7.9, effective in pinpointing vulnerabilities with clear remediation guidance.
- Snyk: Scored 8.1, valued for its extensive library of known vulnerabilities but criticized for frequent noise in results.
Verified Snyk vs Aikido customer testimonials:
Reviews from verified people that have used both Aikido and Snyk. If you want to hear how Aikido stacks up as a Snyk Alternative, read on below.
Aikido is an “effective and fair-priced solution”
Compared to well known competitors like Snyk, Aikido is much more affordable, more complete and most importantly much better at presenting the vulnerabilities that are actually reaching your systems. They use many popular open source libraries to scan your code, as well as propriatary ones, giving you a good mix

Aikido is “a cheaper Snyk Alternative”
We were looking for a cheaper alternative to Snyk and Aikido fills that role fantastically. Good software, easy UI and most important of all very easy to talk to with feedback.
Everything was really simple to set-up and onboarding of team members a breeze.
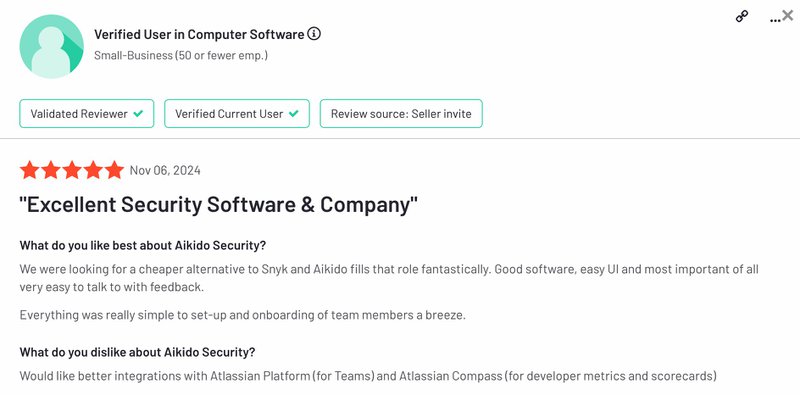
Hopefully, this synopsis of G2 user feedback helps to inform your search for an application security platform. If you are interested in testing Aikido, why not launch now?
Get your first scan results in 32 seconds with no credit card and no strings attached, you can even use demo data for extra security. If you want a more personalized walk-through, you can talk to a human or say “hi” on intercom. We respond in seconds 🤝
if(window.strchfSettings === undefined) window.strchfSettings = {};
window.strchfSettings.stats = {url: "https://aikido-security.storychief.io/en/snyk-vs-aikido-security-g2-reviews-snyk-alternative?id=843344305&type=26",title: "Snyk vs Aikido Security | G2 Reviews Snyk Alternative",siteId: "5823",id: "f8908bf0-c824-4f3f-9bc9-22cac096e678"};
(function(d, s, id) {
var js, sjs = d.getElementsByTagName(s)[0];
if (d.getElementById(id)) {window.strchf.update(); return;}
js = d.createElement(s); js.id = id;
js.src = "https://d37oebn0w9ir6a.cloudfront.net/scripts/v0/strchf.js";
js.async = true;
sjs.parentNode.insertBefore(js, sjs);
}(document, 'script', 'storychief-jssdk'))

Top 10 Software Composition Analysis (SCA) tools in 2025

85% of the code that we use doesn’t come from our own code, it comes from our open-source components and dependencies. This means attackers can know your code better than you do! SCA tools are our best line of defense to keep our open-source supply chain secure.
Software Composition Analysis (SCA) tools, also known as open-source dependency scanning, help us understand the risks we have in our open-source supply chain. From known vulnerabilities, risky licenses or malware hidden in innocent-looking libraries.
Understanding the composition of your open-source supply chain can be very difficult and SCA tools have become an integral part of the application's security programs. However, they often are riddled with false positives and unnecessary noise so we wanted to break down precisely what to look for in a good SCA tool and review 10 of the market leaders in SCA right now.
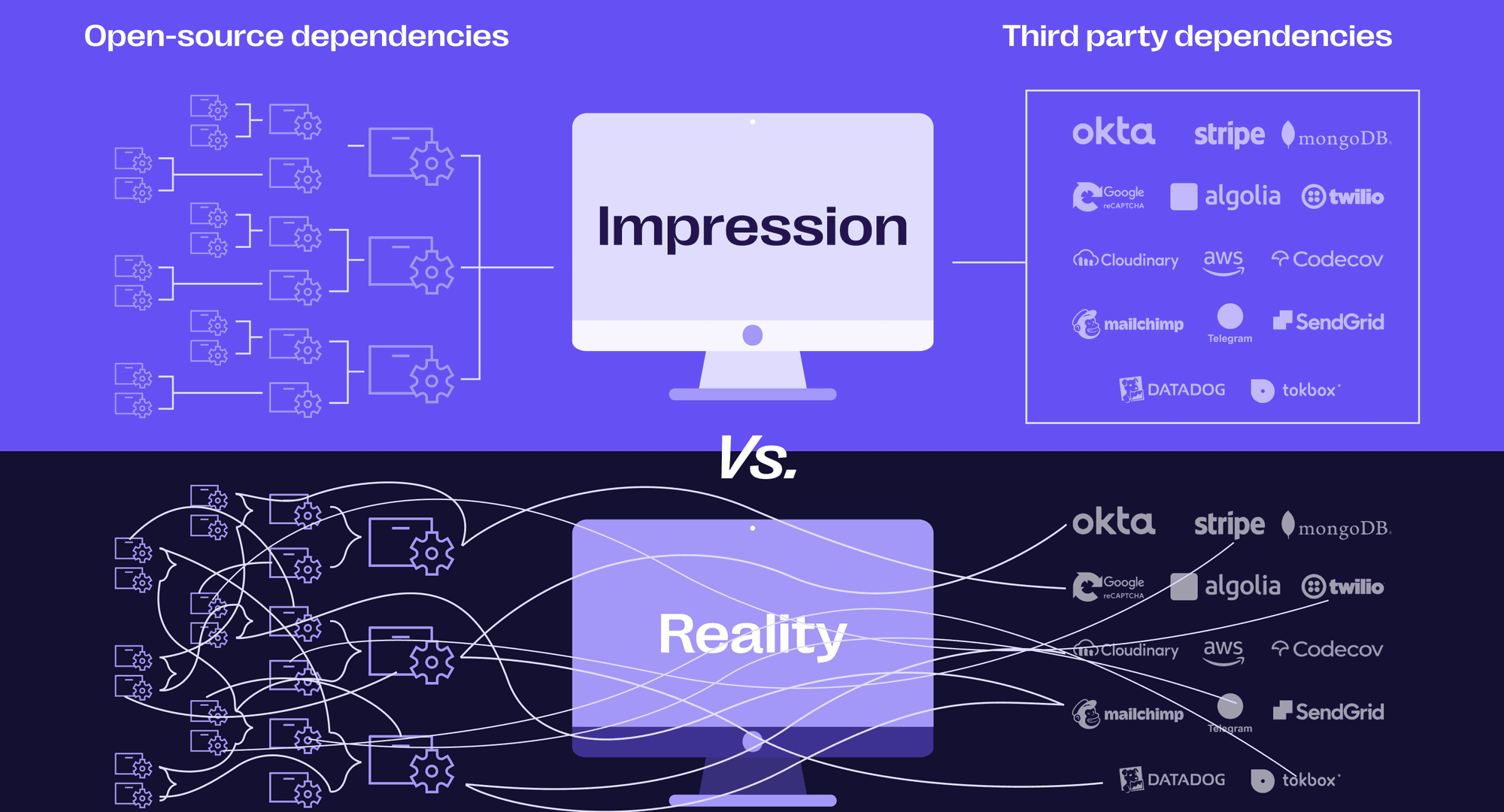
How does Software Composition Analysis Work?
SCA tools provide an ongoing process for detecting vulnerabilities usually by checking our dependencies and versions against known vulnerabilities. Leaders in SCA however will go further and detect packages using high-risk licenses, conduct malware inspection, and even detect when packages are no longer actively maintained. In addition the approach tools take can differ, typically we see 6 different stages within a SCA tool.
- OSS Dependency Scanning
- Scans application codebases, build directories, CI/CD pipelines, and package manager files to identify open-source (OS) dependencies.
- Detects both direct dependencies (explicitly declared) and transitive dependencies (inherited).
- Generating a Software Bill of Materials (SBOM)
- Creates an inventory of all OS components with:
- Component names, versions, locations, suppliers/maintainers
- Associated open-source licenses.
- Often visualizes dependency relationships for better analysis and identifying potential vulnerabilities/conflicts.
- Creates an inventory of all OS components with:
- Vulnerability Assessment
- Compares the SBOM against databases like NVD, CVE, GitHub Advisory, etc.
- Scanning open-source components for malware not declared in databases
- Uses Common Platform Enumeration (CPE) to map components to known vulnerabilities.
- Regularly updated databases ensure new vulnerabilities are flagged, even for older dependencies.
- OSS License Compliance
- Identifies licensing terms for each dependency.
- Examples: GPL (restrictive, requires sharing modifications) vs. MIT (permissive).
- Flags license conflicts or violations of internal organizational policies.
- Identifies licensing terms for each dependency.
- Vulnerability Remediation and Auto-Triaging
- Provides actionable recommendations
- Suggests updates to patched versions (often automatically creating Pull Requests)
- Links to security advisories.
- Offers temporary workarounds.
- Prioritizes vulnerabilities based on severity, exploitability, and runtime impact (auto-triaging).
- Provides actionable recommendations
- Continuous Monitoring and Reporting
- Periodically rescans the codebase for emerging vulnerabilities and updates SBOMs.
- Maintains real-time visibility into OS components, their versions, and associated risks.
Top 10 Industry-proven SCA Tools
(In alphabetical order)
If you are looking for SCA tools and don’t know where to start, here is a list of 10 tools we consider to be industry leaders followed by there core features and any disadvantages.
1. Aikido Security
Aikido Security is a developer-focused no-nonsense security platform that combines 9 different scanners into a single platform protecting you from code to code.
Aikido takes a different approach to open-source dependency scanning by prioritizing vulnerabilities based on real-world risk factors instead of relying solely on CVSS scores and also scans for malware, license risks, and inactive packages.

Key Features:
- Risk-Based Vulnerability Prioritization: Focuses on exploitable issues, considering data sensitivity and vulnerability reachability, reducing noise from irrelevant CVEs.
- Advanced Malware Detection: Identifies hidden malicious scripts and data exfiltration attempts across major ecosystems like NPM, Python, Go, and Rust.
- Reachability Analysis: Uses a robust engine to identify and prioritize actionable vulnerabilities, eliminating false positives and duplicates.
- Automated Remediation Workflows: Integrates with tools like Slack, Jira, and GitHub Actions to automate ticketing, notifications, and security policies.
- Local CLI Scanner: Enables secure, self-hosted scanning for teams handling sensitive data, ensuring compliance with privacy and regulatory standards.
- Developer-Centric Design: Embeds security directly into workflows, offering clear, actionable guidance tailored to the specific impact on codebases.
- Straightforward Pricing: Predictable and cost-effective, with savings of up to 50% compared to competitors.
2. Apiiro
Apiiro combines deep code analysis with runtime behavior monitoring to identify and prioritize exploitable vulnerabilities and open-source risks, providing comprehensive insights and streamlining remediation directly within developer workflows.
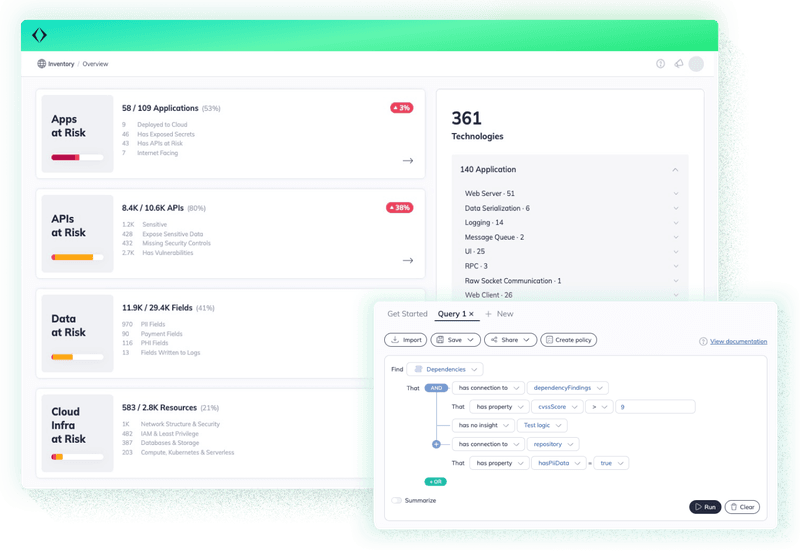
Key Features:
- Comprehensive Risk Analysis: Evaluates open-source risks beyond CVEs, including unmaintained projects, licensing conflicts, and insecure coding practices.
- Penetration Testing Simulations: Confirms the exploitability of vulnerabilities based on runtime context to prioritize critical risks.
- Risk Graph and Control Plane: Maps OSS supply chains and automates workflows, policies, and remediation processes to address risks effectively.
- Extended SBOMs (XBOM): Provides a real-time, graph-based view of dependencies and associated risks, including CI/CD and cloud resources.
- Developer-Centric Remediation: Embeds contextualized alerts and secure version updates into existing developer workflows and tools.
Disadvantages:
- High Cost: Requires a minimum annual contract of $35,400 for 50 seats, which may not be suitable for smaller organizations.
- Complex Onboarding: Advanced features like risk graphing and XBOMs may necessitate a steep learning curve for new users.
3. Arnica
Arnica integrates directly with SCM systems to continuously monitor code changes and dependencies in real-time, providing early detection of vulnerabilities, dynamic inventory management, and actionable remediation guidance to ensure security is embedded into the development lifecycle.

Key Features:
- Pipelineless SCA: Eliminates complex pipeline setups by natively integrating with tools like GitHub, GitLab, and Azure DevOps to scan every commit in real-time.
- Dynamic Dependency Inventory: Maintains an up-to-date inventory of all external packages, licenses, and associated risks.
- Exploitability Prioritization: Correlates OpenSSF scorecards and EPSS threat intelligence to calculate exploitability risk scores for each vulnerability.
- Contextual Alerting: Delivers detailed, prescriptive alerts to relevant stakeholders with step-by-step remediation guidance, including one-click automated fixes.
- Seamless Feedback Loop: Provides immediate security feedback to developers, fostering early and continuous vulnerability management.
Disadvantages:
- Limited Free Features: Advanced functionalities require paid plans, starting at $8 per identity per month.
- Scaling Costs: Costs increase with the number of identities, which may be a concern for large teams or organizations.
4. Cycode
Cycode provides end-to-end visibility into open-source vulnerabilities and license violations by scanning application code, CI/CD pipelines, and infrastructure, offering real-time monitoring, automated SBOM generation, and scalable remediation directly integrated into developer workflows.

Key Features:
- Comprehensive Scanning: Analyzes application code, build files, and CI/CD pipelines for vulnerabilities and license violations.
- Real-Time Monitoring: Uses a knowledge graph to identify deviations and potential attack vectors as they occur.
- SBOM Management: Generates up-to-date SBOMs in SPDX or CycloneDX formats for all dependencies.
- Integrated Remediation: Provides CVE context, suggested upgrades, one-click fixes, and automated pull requests to accelerate patching.
- Scalable Fixes: This enables addressing vulnerabilities across repositories in a single action.
Disadvantages:
- Pricing Transparency: Requires direct contact for pricing, with estimates suggesting $350 per monitored developer annually.
- Cost for Larger Teams: Pricing may become prohibitive for organizations with many developers.
5. Deep Factor
DeepFactor combines static scanning with live runtime monitoring to generate comprehensive SBOMs, map dependencies, and identify exploitable risks by analyzing real-world execution patterns and runtime behaviors, offering a contextualized view of vulnerabilities to streamline remediation.
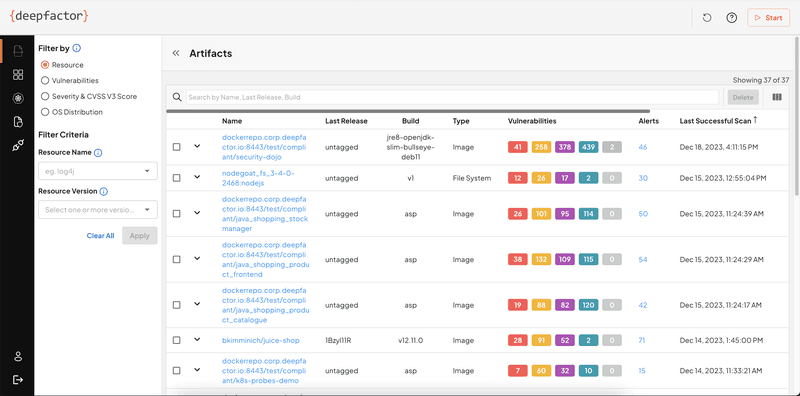
Key Features:
- Runtime Reachability SCA: Tracks whether vulnerabilities are exploitable by analyzing executed code paths, control flows, and stack traces.
- Dynamic SBOM Generation: Identifies all dependencies, including undeclared "phantom" components, by combining static and runtime analysis.
- Customizable Security Policies: Allows organizations to define unique conditional rules and triggers based on their specific security needs.
- Intelligent Alert Correlation: Consolidates related issues into actionable alerts with detailed context, reducing triage noise.
- Granular Runtime Insights: Observes application behavior across file operations, memory usage, network activity, and more.
Disadvantages:
- Pricing: Costs can add up quickly for larger teams, with the all-in-one plan at $65/developer/month.
- Limited Language Support: Runtime reachability analysis currently supports a subset of languages (PHP, Kotlin, Go, Ruby, Scala), which may not cover all use cases.
6. Endor Labs
Endor Labs enhances SCA scanning by inspecting source code to build dynamic SBOMs, identify critical vulnerabilities, and detect insecure coding patterns, malware, and inactive dependencies, enabling DevSecOps teams to focus on the most exploitable risks with actionable insights and regulatory compliance support.

Key Features:
- Granular Dependency Analysis: Maps all declared and "phantom" dependencies through source code inspection, not just manifest files.
- Reachability Analysis: Identifies vulnerabilities realistically exploitable in the application’s context to reduce noise.
- Endor Score: Provides a comprehensive health assessment of OSS packages, factoring in security history, community support, and maintenance.
- Automated SBOM and VEX Reports: Continuously updates dependency inventories and vulnerability classifications with in-depth reachability context.
- Advanced Detection Capabilities: Includes rules engines to flag malware, insecure patterns, dependency sprawl, and license violations.
Disadvantages:
- High Entry Cost: Paid plans start at $10,000 annually, making it less accessible for smaller organizations.
- Complexity for New Users: The comprehensive features and in-depth analysis may require onboarding time for new teams.
7. Oligo Security
Oligo adopts a unique approach to SCA by monitoring libraries at runtime, in both testing and production, to detect vulnerabilities that traditional scanners miss. Oligo offers actionable fixes based on application context and environment. By leveraging an extensive knowledge base of library behavior profiles and real-time monitoring, Oligo identifies zero-day vulnerabilities, improper library usage, and runtime-specific threats, ensuring DevSecOps teams address critical issues efficiently.
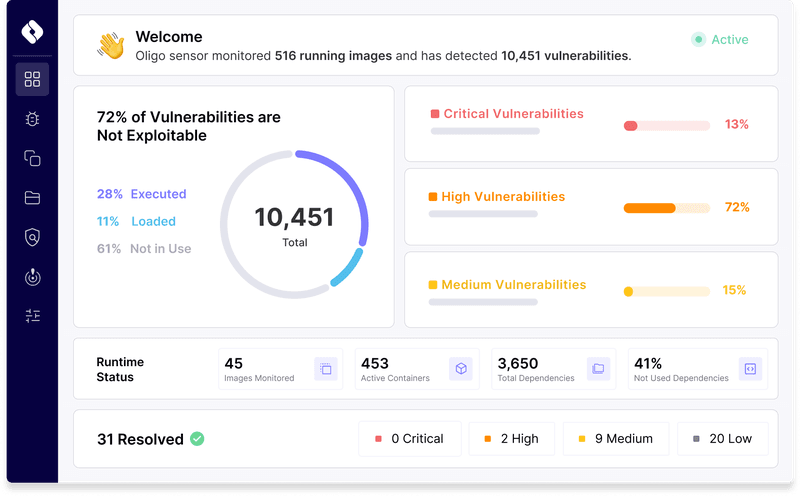
Key Features:
- Runtime Monitoring: Tracks library behavior during testing and production to detect deviations and vulnerabilities.
- eBPF-Based Profiling: Utilizes Linux kernel-level monitoring for unmatched visibility into runtime behavior.
- Automated Policies and Triggers: Customizable security workflows and real-time alerts via tools like Slack and Jira.
- Zero-Day Vulnerability Detection: Identifies threats before they are publicly known, preventing zero-day attacks.
- Contextual Vulnerability Prioritization: Considers environment and library execution state to prioritize threats effectively.
Disadvantages:
- Pricing Transparency: Requires a demo to access pricing details; no self-serve or standardized pricing information is available.
- Platform Limitations: Primarily Linux-focused due to reliance on eBPF technology.
8. Semgrep
Semgrep is a comprehensive supply chain security platform that scans across the development workflow, leveraging lightweight pattern matching and reachability analysis to detect vulnerabilities and anti-patterns directly exploitable in your code, while offering customizable rules and real-time dependency visibility.

Key Features:
- End-to-End Scanning: Monitors IDEs, repositories, CI/CD pipelines, and dependencies for security threats and anti-patterns.
- Reachability Analysis: Identifies if flagged vulnerabilities are actively exploitable in your application, reducing unnecessary noise.
- Dependency Search: Provides live, queryable streams of third-party packages and versions for real-time threat response and upgrade planning.
- Semgrep Registry: Features over 40,000 pre-built and community-contributed rules, with options for custom rule creation.
- Broad Language Support: Supports 25+ modern programming languages, including Go, Java, Python, JavaScript, and C#.
- Seamless Integrations: Works out-of-the-box with GitHub, GitLab, and other popular version control systems.
Disadvantages:
- Pricing for Larger Teams: Costs escalate quickly for mid-sized and large teams ($110/contributor/month for 10+ contributors).
- Customization Complexity: Writing and managing custom rules may require additional effort for less experienced teams.
9. Snyk
Snyk has become the gold standard for traditional SCA tools, it creates detailed dependency trees, identifies nested dependencies, and creates prioritized remediation efforts based on real-world risk factors and exploitability. Snyk fits into the developer workflows with dashboard, CLI / IDE tools, provides actionable fixes, and helps ensure open-source license compliance.

Key Features:
- Dependency Tree Mapping: Builds hierarchical graphs to detect vulnerabilities in direct and transitive dependencies and trace their impact.
- Proprietary Priority Scoring: Ranks vulnerabilities based on exploitability, context, and potential impact, ensuring focus on critical threats.
- Snyk Advisor: Assesses over 1 million open-source packages for security, quality, and maintenance to help developers choose the best dependencies.
- Vulnerability Database: Maintains a robust database of 10+ million open-source vulnerabilities, manually vetted for accuracy and actionable insights.
- Seamless Integration: Works with popular version control systems, CI/CD pipelines, and IDEs to scan code and dependencies in real time.
- Customizable Policies: Allows organizations to enforce specific rules for vulnerability handling and license compliance.
Disadvantages:
- Cost for Advanced Features: While the free plan is basic, advanced features for larger teams require higher-tier plans, which can be costly.
- Manual Verification Dependency: Reliance on manual vetting for vulnerabilities may delay updates for newly discovered threats.
10. Socket Security
Socket leverages deep package inspection and runtime behavior analysis to proactively detect supply chain threats, zero-day vulnerabilities, and anomalies in open-source dependencies, ensuring comprehensive protection beyond traditional SBOM-based scanning.
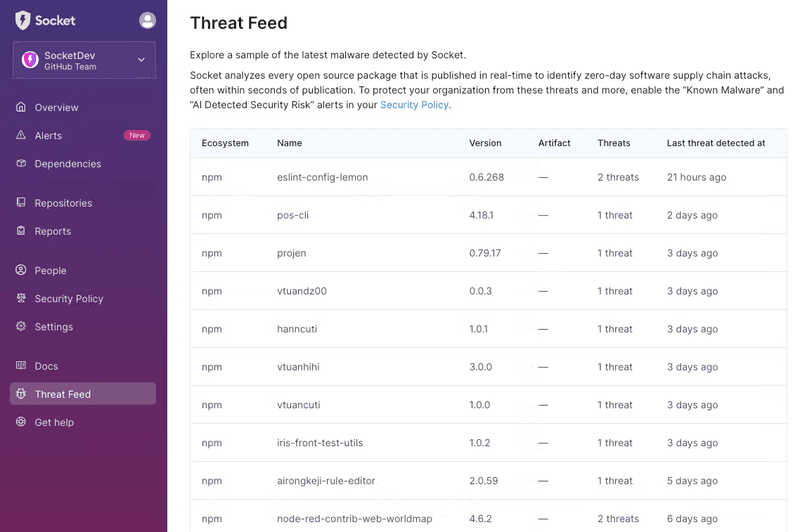
Key Features:
- Deep Package Inspection: Monitors dependencies' runtime behavior, including resource interactions and permission requests, to detect risky behaviors.
- Proactive Threat Detection: Identifies zero-day vulnerabilities, typosquatting risks, and supply chain attacks before they’re publicly disclosed.
- Pull Request Integration: Automatically scans dependencies with every pull request and provides actionable GitHub comments, ensuring early risk mitigation.
- Dependency Overview: Offers insights into direct and transitive dependencies, providing a complete dependency graph with critical details and links.
- Maintenance Risk Assessment: Evaluates maintainer activity, codebase updates, and social validation to flag potential risks in OSS packages.
Disadvantages:
- Language Support: Limited to JavaScript, Python, and Go dependencies, which may restrict usage for teams working in other languages.
Choosing The Right OSS Dependency Scanner
Choosing the right SCA tool is going to depend on the specific needs of your project and the technology it uses. It is important to note that SCA is only one part of a comprehensive application security plan and using a stand-alone SCA tool will mean needing to integrate with multiple different vendors. All-in-one solutions like Aikido security are not just attractive in

Want to see Aikido in action? Sign up to scan your repos and get your first SCA results in less than 2 minutes.

3 Key Steps to Strengthen Compliance and Risk Management

A robust security strategy is no longer a nice-to-have. It's essential to remain competitive and trustworthy in the market. Security teams are under constant pressure to quickly address vulnerabilities and maintain compliance, all while scaling business operations. In this blogpost, Roeland Delrue and Sonali Samantaray, co-founder of Aikido Security and Sr Solutions Engineer at Sprinto, respectively, share their expertise in scaling security solutions that balance risk, growth, and compliance requirements. As leaders in vulnerability management and compliance automation, they provide actionable insights to help organizations secure their growth path.
1. Stay on Top of Risks
As organizations scale, their risk profile grows. With each new process, customer, or data touchpoint, the security landscape expands, making it more difficult for teams to identify, assess, and prioritize vulnerabilities. Without an integrated, real-time approach, risks can accumulate and the potential for damage - reputational or financial - can multiply. One small misconfiguration or overlooked vulnerability can expose millions of sensitive data points or disrupt operations.
As Roeland notes, "Security isn’t just a safeguard - it's a crucial part of your business’s ability to grow and earn trust. If you’re not managing risk, your customers will notice - and they may decide to go elsewhere. Focusing on the right security efforts helps you build that trust and drive business growth.”
By automating security tools with integrated compliance management, organizations can streamline risk tracking, reduce manual remediation, and ensure real-time compliance - enabling teams to focus on proactive security measures rather than reactive patchwork. Automated tools help teams by providing real-time visibility, reducing alert fatigue, and simplifying compliance, creating a foundation that supports proactive risk management.
2. Prioritize Effective Remediation
Managing a growing list of vulnerabilities and risks can quickly become overwhelming. When faced with dozens or even hundreds of security alerts, teams often fall into a cycle of alert fatigue - where the sheer volume of notifications desensitizes them to the risks at hand. This can lead to analysis paralysis, where decision-making becomes harder and progress slows down because there are too many issues to address at once. According to Roeland, this volume can undermine the effectiveness of remediation efforts.
"When you overwhelm your team with hundreds of issues, the chances of them resolving any of them effectively drops significantly. It’s all about prioritizing the most critical problems, so your team can focus on what truly matters. This focused approach not only reduces alert fatigue but ensures that the issues addressed are the ones that will make the biggest impact," says Roeland.
To avoid this pitfall, it's critical to focus your security efforts on what matters most. Tools that highlight the most pressing issues - not just the most numbers - help teams make meaningful progress. With clear priorities for remediation, organizations can reduce the risk of critical vulnerabilities slipping through the cracks.
Without the right focus, teams end up in a constant reactive state. But a targeted approach - where you can address high-risk issues right away- allows teams to get out of the reactive loop and spend time on proactive security measures.
"Without the right focus," Sonali explains, "teams end up in a constant reactive state. But a targeted approach - where you can address high-risk issues right away- allows teams to get out of the reactive loop and spend time on proactive security measures." By prioritizing alerts, reducing false positives, and deploying specialized security tools, organizations can avoid the whack-a-mole cycle of constantly addressing low-priority issues while missing the big ones. By focusing remediation efforts on high-impact vulnerabilities, security teams can effectively manage risk and focus on other high-value tasks, such as developing new features or refining product offerings.
3. Centralize Your Security Posture
With so many components, it's easy for security data to become siloed across platforms and teams. Siloed data creates blind spots in your security posture, making it difficult for security teams to act quickly and efficiently. This disconnect can lead to compliance failures, security breaches, and delays that prevent your organization from scaling securely.
"Having everything in one place isn't just about convenience," says Roeland. "It's about getting a complete, real-time picture of your risk and compliance landscape. A centralized system makes it easier to connect the dots, identify gaps, and prioritize resources effectively."
Having everything in one place isn't just about convenience. It's about getting a complete, real-time picture of your risk and compliance landscape. A centralized system makes it easier to connect the dots, identify gaps, and prioritize resources effectively.
A centralized security posture brings all of these elements - vulnerability management, compliance tracking, and ongoing risk assessment- into a unified view. This approach enables security teams to see their entire landscape at once, identify and address gaps in real time, and create a cohesive system that's always audit-ready. By combining a vulnerability-focused solution like Aikido with a compliance-focused platform like Sprinto, organizations can streamline their security practices, reduce manual reporting, and ensure that compliance and security work hand in hand rather than in isolation.
The Path Forward
Navigating today's complex security landscape requires an approach that is both proactive and focused. By staying on top of risks, prioritizing effective remediation, and centralizing their security posture, organizations can not only improve their security but also enhance their ability to scale safely and confidently. As Roeland aptly states, "Security is not a one-time project. It's an ongoing commitment to your customers and your growth." Investing in these steps isn't just about keeping up with security standards - it's about enabling business growth while keeping customer trust at the forefront.